Python Practice Problems for Beginner Coders
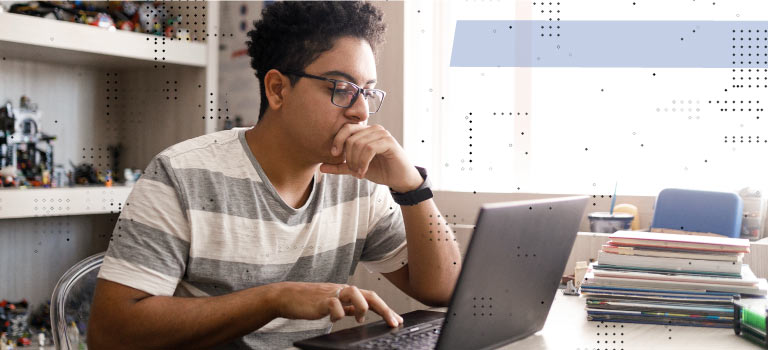
From sifting through Twitter data to making your own Minecraft modifications, Python is one of the most versatile programming languages at a coder’s disposal. The open-source, object-oriented language is also quickly becoming one of the most-used languages in data science.
According to the Association for Computing Machinery, Python is now the most popular introductory language at universities in the United States.
To help readers practice the Python fundamentals, datascience@berkeley gathered six coding problems, including some from the W200: Introduction to Data Science Programming course. The questions below cover concepts ranging from basic data types to object-oriented programming using classes.
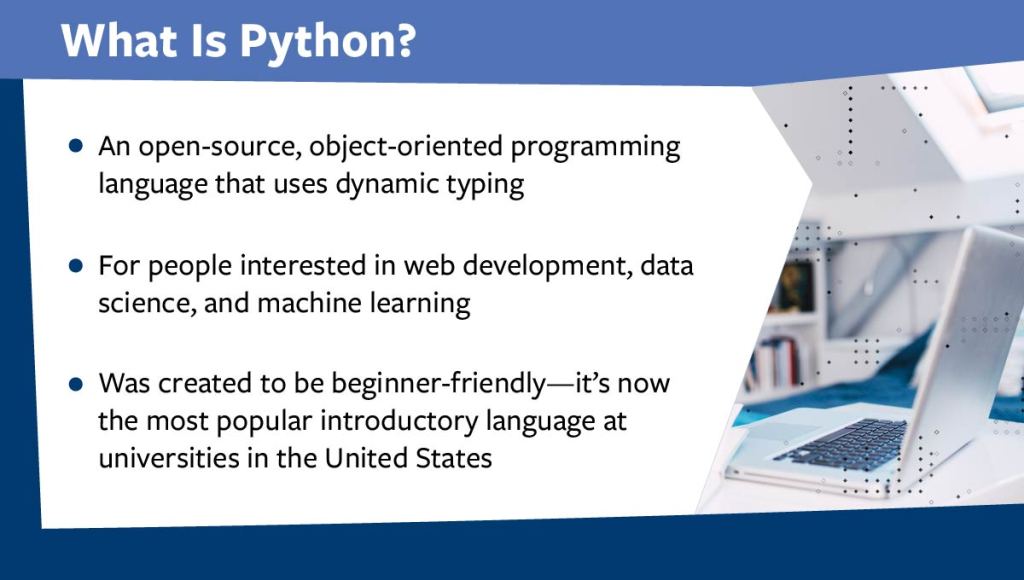
Are You Ready to Start Your Python Practice?
Consider the following questions to make sure you have the proper prior knowledge and coding environment to continue.
How much Python do I need to already know?
This problem set is intended for people who already have familiarity with Python (or another language) and data types. Each problem highlights a few different skills, and they gradually become more complicated. To learn more about the different fundamentals tested here, use the Resources section accompanying each question.
Readers who want to learn more about Python before beginning can access the following tools:
Where do I write my code?
datascience@berkeley created a Google Colab notebook as a starting point for readers to execute their code. Google Colab is a free computational environment that allows anyone with an Internet connection to execute Python code via the browser.
For a more thorough introduction to Google’s Colab notebooks and how to use them, check out this guide to Getting Started with Google Colab from Towards Data Science.
You can also execute code using other systems, such as a text editor on your local machine or a Jupyter notebook.
My answers don’t look the same as the provided solutions. Is my code wrong?
The solutions provided are examples of working code to solve the presented problem. However, just like multiple responses to the same essay prompt will look different, every solution to a coding problem can be unique.
As you develop your own coding style, you may prefer different approaches. Instead of worrying about matching the example code exactly, focus on making sure your code is accurate, concise, and clear.
I’m ready, let’s go!
Below are links to the Google Colab notebooks created by datascience@berkeley that you can use to create and test your code.
Python Practice Problems:
QUESTIONS
This notebook contains the questions and space to create and test your code. To use it, save a copy to your local drive.
Python Practice Problems:
SOLUTIONS
This notebook contains the questions and corresponding solutions.
Python Exercises
1. Fly Swatting: Debugging and String Formatting Exercise
The following code chunk contains errors that prevent it from executing properly. Find the “bugs” and correct them.
def problem_one():
capitals =
“Maryland” == “Annapolis”
“California”: Sacramento,
“New York”: “Albany,
“Utah'': “Salt Lake City”,
“Alabama”: “Montgomery”
}
for state, city in capitals.items()
print(f”The capital of {state) is {‘city’}.”
Once fixed, it should return the following output:
The capital of Maryland is Annapolis.
The capital of California is Sacramento.
The capital of New York is Albany.
The capital of Utah is Salt Lake City.
The capital of Alabama is Montgomery.
Python Debugging and String Formatting Resources
2. That’s a Wrap: Functions and Wrapped Functions Exercise
Write a function multiply()
that takes one parameter, an integer x
. Check that x
is an integer at least three characters long. If it is not, end the function and print a statement explaining why. If it is, return the product of x
’s digits.
Write another function matching()
that takes an integer x
as a parameter and “wraps” your multiply()
function. Compare the results of the multiply()
function on x
with the original x
parameter and return a sorted list of any shared digits between the two. If there are none, print “No shared digits!”
Your code should reproduce the following examples:
>multiply(1468)
192
>multiply(74)
This integer is not long enough!
>matching(2789475)
[2,4]
Python Functions Resources
3. Show Me the Money: Data Types and Arithmetic Exercise
Write code that asks a user to input a monetary value (USD). You can assume the user will input an integer or float with no special characters. Return a print statement using string formatting that states the value converted to Euros (EUR), Japanese Yen (JPY), and Mexican Pesos (MXN). Your new values should be rounded to the nearest hundredth.
Your code should reproduce the following examples:
>Input a value to convert from $USD: 189
189 USD = 160.65 EUR = 20,909.07 JPY = 3,783.78 MXN
>Input a value to convert from $USD: 17.82
17.82 USD = 15.15 EUR = 1,971.43 JPY = 356.76 MXN
Hint: To match the exact values from the example, you can use currency conversion ratios from the time of publication. As of July 6, 2021, $1 USD is equivalent to 0.85 EUR, 110.63 JPY, and 20.02 MXN.
Python Data Types and Arithmetic Resources:
4. What’s in a Name: String Slicing and If/Else Statements Exercise
Write a script that prompts the user for a name (assume it will be a one-word string). Change the string to lowercase and print it out in reverse, with only the first letter of the reversed word in uppercase. If the name is the same forward as it is backward, add an additional print statement on the next line that says “Palindrome!”
Your code should reproduce the following examples:
>Enter your name: Paul
Luap
>Enter your name: ANA
Ana
Palindrome!
Hint: Use s.lower()
and s.upper()
, as appropriate.
Python String and If/Else Statement Resources:
5. Adventures in Loops: “For” Loops and List Comprehensions Exercise
Save the paragraph below — from Alice’s Adventures in Wonderland — to a variable named text
. Write two programs using “for” loops and/or list comprehensions to do the following:
- Create a single string that contains the second-to-last letter of each word in
text
, sorted alphabetically and in lowercase. Save it to a variable namedletters
and print. If a word is less than two letters in length, use the single character available. - Find the average number of characters per word in
text
, rounded to the nearest hundredth. This value should exclude special characters, such as quotation marks and semicolons. Save it to a variable namedavg_chars
and print.
text = “Alice was beginning to get very tired of sitting \
by her sister on the bank, and of having nothing to do: \
once or twice she had peeped into the book her sister \
was reading, but it had no pictures or conversations in \
it, ‘and what is the use of a book,’ thought Alice, \
‘without pictures or conversations?’”
Python “for” Loop and List Comprehensions Resources:
6. The Drones You’re Looking For: Classes and Objects
Make a class named Drone
that meets the following requirements:
- Has an attribute named
altitude
that stores the altitude of theDrone
. Use a property to set thealtitude
attribute and make it hidden. - Create getter and setter methods for the
altitude
attribute. Your setter method should return an exception if the passedaltitude
is negative. - Has a method named
ascend
that causes theDrone
to ascend to a passed altitude change. - Has an attribute named
ascend_count
that stores the number of ascents theDrone
has done. - Has a method named
fly
that returns theDrone
’s currentaltitude
.
Your code should mimic this sample output:
> d1 = Drone(100)
> d1.fly()
The drone is flying at 100 feet.
> d1.altitude = 300
> d1.fly()
The drone is flying at 300 feet.
> d1.ascend(100)
> d1.fly()
The drone is flying at 400 feet.
> d1.ascend_count
1
Python Classes and Objects Resources:
7. Top of the Class: Dictionaries and “While” Loops Exercise
Write a program that lets the user create and manage a gradebook for their students. Create a Gradebook
class that begins with an empty dictionary. Use helper functions inside the class to run specific tasks at the user’s request. Use the input()
method to ask users what task they would like to complete. The program should continue to prompt the user for tasks until the user decides to quit. Your program must allow the user to do the following:
Add student
: Creates a new entry in a dictionary called `gradebook.` The user will input the key (a student’s name) and the value (a list of grades). You can assume grades will be integers separated by commas and no spaces.Add grades
: Adds additional grades to an existing student’sgradebook
entry.View gradebook
: Prints the entiregradebook
.Calculate averages
: Prints each student’s unweighted average grade, rounded to the nearest hundredth.Quit
: Ends the program.
Your code should reproduce the following example:
>What would you like to do?: Add student
>Enter student name: Natalie
A new student! Adding them to the gradebook...
>Enter student grade(s): 87,82
New entry complete!
>What would you like to do?: View gradebook
{'natalie': [87.0, 82.0]}
>What would you like to do?: Calculate averages
natalie: 84.50
>What would you like to do?: Quit
End of program
Hint: Use a “while” loop to run the program while the input()
response is anything other than “quit.” Within the “while” loop, set up if/else statements to manage each potential response.
Python Dictionaries and “While” Loop Resources:
Additional Python Coding Exercises
Looking for more Python practice? Here are some additional problem sets to work on fundamental coding skills:
Advent of Code
This site hosts a yearly advent calendar every December, with coding challenges that open daily at midnight PST. Coders can build their own small group competitions or compete in the overall one. Past years’ problems are available for non-competitive coding.
Eda Bit
Thousands of Python challenges that use an interactive interface to run and check code against the solutions. Users can level up and sort by difficulty.
PracticePython.org
These 36 exercises test users’ knowledge of concepts ranging from input()
to data visualization.
Python exercises, w3resource
A collection of hundreds of Python exercises organized by concept and module. This set also includes a section of Pandas data exercises.
Created by datascience@berkeley, the online Master of Information and Data Science from UC Berkeley